ListView は、指定したモデルのオブジェクトのリストを取得してテンプレートに渡し、それを一覧表示するために使われます。
モデルの定義
test_app/models.py に今回は以下のように記述しました。
from django.db import models # Create your models here. from django.conf import settings class Book(models.Model): title = models.CharField(verbose_name = 'タイトル', max_length=64) description = models.TextField(verbose_name = '本の説明', blank=True) author = models.CharField(verbose_name = '著者', max_length=64) creator = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE, null=True, blank=True, verbose_name = '作成者') def __str__(self): return self.title
View
test_app/views.py を以下のように記述します。
# test_app/views.py from django.views.generic import ListView # ShareCode を使うためにインポート from .models import ShareCode class ShareCodeListView(ListView): model = ShareCode template_name = "test_app/top_page.html" context_object_name = "codes"
主なクラス属性
クラス属性 | 説明 | 使用例 |
---|---|---|
model | 対象となるモデルを指定する | model = Book |
template_name | 使用するテンプレートファイル名を指定する | template_name = “test_app/book_list.html” |
context_object_name | テンプレート内で使うオブジェクト名を指定する | context_object_name = “books” |
paginate_by | 1ページあたりの表示件数を指定する | paginate_by = 10 |
ordering | 並び順を指定する(昇順/降順) | ordering = [“-published_date”] |
queryset | 独自のクエリセットを指定する | queryset = Book.objects.filter(published=True) |
allow_empty | 空のリストでも表示を許可するかどうか | allow_empty = True |
extra_context | テンプレートに渡す追加の文脈(コンテキスト)を辞書で指定する | extra_context = {“title”: “本一覧”} |
template_name_suffix | テンプレートファイル名のサフィックス(末尾)を指定する | template_name_suffix = “_overview” |
ルーティングの設定
URLにBookListViewを記載
from django.urls import path from .views import * urlpatterns = [ # /にアクセスした時、BookListViewを呼び出す path("", BookListView.as_view(), name="book_list"), ]
テンプレート
Viewからbooksという名前で送られてくるので、それをforで取得します。
<!-- test_app/templates/test_app/top_page.html --> <!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <title>トップページ</title> </head> <body> <h1>ようこそ、トップページへ!</h1> {% for book in books %} <li>タイトル:{{ book.title }}</li> <li>本の説明:{{ book.description }}</li> <li>著者:{{ book.author }}</li> <li>記事の作成者:{{ book.creator }}</li> <br> {% endfor %} </body> </html>
マイグレーションし、テーブルにデータを追加後、「http://127.0.0.1:8000/」にアクセスすると以下のように表示されます。
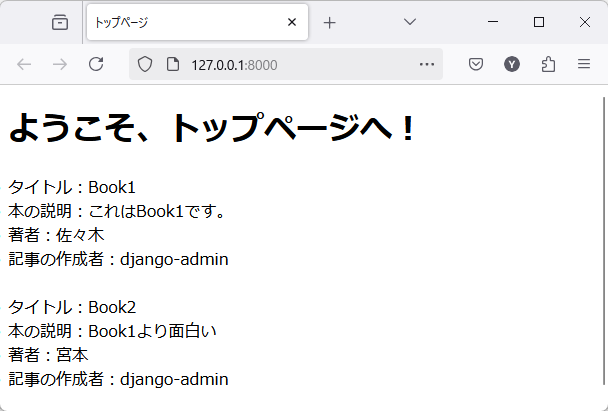
コメント